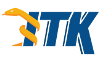 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkSmartPointer_h
20 #define itkSmartPointer_h
24 #include <type_traits>
25 #include "itkConfigure.h"
50 template <
typename TObjectType>
73 template <typename T, typename = typename EnableIfConvertible<T>::type>
84 p.m_Pointer =
nullptr;
88 template <typename T, typename = typename EnableIfConvertible<T>::type>
92 p.m_Pointer =
nullptr;
110 explicit operator bool() const noexcept {
return m_Pointer !=
nullptr; }
188 #if !defined(ITK_LEGACY_REMOVE)
201 other.m_Pointer = tmp;
208 template <
typename T>
232 template <
class T,
class TU>
236 return (l.GetPointer() == r.GetPointer());
242 return (l.GetPointer() ==
nullptr);
248 return (
nullptr == r.GetPointer());
253 template <
class T,
class TU>
257 return (l.GetPointer() != r.GetPointer());
263 return (l.GetPointer() !=
nullptr);
269 return (
nullptr != r.GetPointer());
275 template <
class T,
class TU>
279 return (l.GetPointer() < r.GetPointer());
283 template <
class T,
class TU>
287 return (l.GetPointer() > r.GetPointer());
291 template <
class T,
class TU>
295 return (l.GetPointer() <= r.GetPointer());
299 template <
class T,
class TU>
303 return (l.GetPointer() >= r.GetPointer());
306 template <
typename T>
314 template <
typename T>
void Swap(SmartPointer &other) noexcept
SmartPointer(const SmartPointer< T > &p) noexcept
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator<=(const Index< VDimension > &one, const Index< VDimension > &two)
SmartPointer(ObjectType *p) noexcept
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
void swap(Array< T > &a, Array< T > &b)
SmartPointer(SmartPointer< ObjectType > &&p) noexcept
SmartPointer & operator=(SmartPointer r) noexcept
Implements transparent reference counting.
ObjectType * Print(std::ostream &os) const
constexpr SmartPointer() noexcept=default
typename std::enable_if< std::is_convertible_v< T *, const Self * > > EnableIfConvertible
ObjectType * GetPointer() const noexcept
void UnRegister() noexcept
ObjectType & operator*() const noexcept
bool operator>=(const Index< VDimension > &one, const Index< VDimension > &two)
ObjectType * operator->() const noexcept
bool operator>(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
bool IsNull() const noexcept
SmartPointer(SmartPointer< T > &&p) noexcept
bool operator!=(const Index< VDimension > &one, const Index< VDimension > &two)
SmartPointer & operator=(std::nullptr_t) noexcept
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
bool IsNotNull() const noexcept
constexpr SmartPointer(std::nullptr_t) noexcept
ObjectType * get() const noexcept