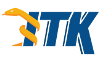 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkSimplexMeshVolumeCalculator_h
19 #define itkSimplexMeshVolumeCalculator_h
53 template <
typename TInputMesh>
124 using PointIdIterator =
typename SimplexPolygonType::PointIdIterator;
133 m_Mesh->GetPoint(*it, &p);
134 center += p.GetVectorFromOrigin();
142 m_CenterMap->InsertElement(cellId, center);
163 CellInterfaceVisitorImplementation<InputPixelType, InputCellTraitsType, SimplexPolygonType, SimplexCellVisitor>;
177 itkGetConstMacro(Volume,
double);
180 itkGetConstMacro(
Area,
double);
186 PrintSelf(std::ostream & os,
Indent indent)
const override;
212 double m_Volume{ 0.0 };
213 double m_VolumeX{ 0.0 };
214 double m_VolumeY{ 0.0 };
215 double m_VolumeZ{ 0.0 };
216 double m_Area{ 0.0 };
220 double m_Wxyz{ 0.0 };
233 #ifndef ITK_MANUAL_INSTANTIATION
234 # include "itkSimplexMeshVolumeCalculator.hxx"
SmartPointer< Self > Pointer
SmartPointer< const Self > ConstPointer
typename InputMeshType::PointsContainer InputPointsContainer
typename InputPointsContainer::ConstPointer InputPointsContainerPointer
ImageBaseType::SpacingType VectorType
ImageBaseType::PointType PointType
typename InputPointType::VectorType VectorType
typename CellMultiVisitorType::Pointer CellMultiVisitorPointer
Represents a polygon in a Mesh.
Control indentation during Print() invocation.
typename PointMapType::Pointer PointMapPointer
A wrapper of the STL "map" container.
unsigned int GetNumberOfPoints() const override
typename SimplexVisitorInterfaceType::Pointer SimplexVisitorInterfacePointer
Light weight base class for most itk classes.
A template class used to implement a visitor object.
typename InputMeshType::CellType SimplexCellType
typename InputMeshType::NeighborListType InputNeighbors
typename InputMeshType::PointType InputPointType
PointIdIterator PointIdsEnd() override
The class represents a 2-simplex mesh.
void Visit(IdentifierType cellId, SimplexPolygonType *poly)
visits all polygon cells and compute the cell centers
A templated class holding a n-Dimensional covariant vector.
typename InputMeshType::MeshTraits::CellTraits InputCellTraitsType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Adapted from itkSimplexMeshToTriangleFilter to calculate the volume of a simplex mesh using the baryc...
typename InputMeshType::NeighborListType::iterator InputNeighborsIterator
void SetMesh(InputMeshPointer mesh)
typename InputPointsContainer::ConstIterator InputPointsContainerIterator
typename InputMeshType::Pointer InputMeshPointer
Base class for most ITK classes.
PointMapPointer GetCenterMap()
typename SimplexCellType::MultiVisitor CellMultiVisitorType
PointIdIterator PointIdsBegin() override
typename InputMeshType::ConstPointer InputMeshConstPointer
PointMapPointer m_CenterMap
SizeValueType IdentifierType
unsigned long SizeValueType
typename InputMeshType::PixelType InputPixelType