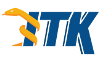 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkRegionBasedLevelSetFunctionSharedData_h
19 #define itkRegionBasedLevelSetFunctionSharedData_h
64 template <
typename TInputImage,
typename TFeatureImage,
typename TSingleData>
75 static constexpr
unsigned int ImageDimension = TFeatureImage::ImageDimension;
unsigned int m_NumberOfNeighbors
SmartPointer< Self > Pointer
typename FeatureImageType::IndexType FeatureIndexType
SmartPointer< const Self > ConstPointer
void SetKdTree(KdTreePointer kdtree)
std::list< unsigned int > ListPixelType
ListImagePointer m_NearestNeighborListImage
Vector< SpacingValueType, VImageDimension > SpacingType
typename InputImageType::ConstPointer InputImageConstPointer
unsigned int m_FunctionCount
SmartPointer< Self > Pointer
ImageBaseType::PointType PointType
A templated class holding a n-Dimensional vector.
typename ListImageType::SizeType ListSizeType
void SetFunctionCount(const unsigned int n)
TInputImage InputImageType
typename ListSizeType::SizeValueType ListSizeValueType
ImageBaseType::SizeType SizeType
This class is the native implementation of the a Sample with an STL container.
typename FeatureSizeType::SizeValueType FeatureSizeValueType
typename InputSizeType::SizeValueType InputSizeValueType
typename TreeGeneratorType::Pointer TreePointer
virtual void PopulateListImage()=0
SmartPointer< Self > Pointer
typename TreeType::Pointer KdTreePointer
typename TreeGeneratorType::KdTreeType TreeType
typename InputIndexType::IndexValueType InputIndexValueType
typename FeatureImageType::PixelType FeaturePixelType
Point< PointValueType, VImageDimension > PointType
typename InputImageType::Pointer InputImagePointer
typename FeatureImageType::SizeType FeatureSizeType
Index< VImageDimension > IndexType
~RegionBasedLevelSetFunctionSharedData() override=default
ImageBaseType::IndexType IndexType
RegionBasedLevelSetFunctionSharedData()
typename ListImageType::ConstPointer ListImageConstPointer
typename ListImageType::SpacingType ListSpacingType
Light weight base class for most itk classes.
typename InputImageType::SpacingType InputSpacingType
typename FeatureImageType::Pointer FeatureImagePointer
typename InputImageType::IndexType InputIndexType
void AllocateListImage(const FeatureImageType *featureImage)
ImageBaseType::RegionType RegionType
typename InputImageType::PixelType InputPixelType
KdTree< TSample > KdTreeType
typename InputImageType::PointType InputPointType
ImageRegion< VImageDimension > RegionType
typename InputImageType::SizeType InputSizeType
typename ListImageType::IndexType ListIndexType
typename FeatureImageType::PointType FeaturePointType
void CreateHeavisideFunctionOfLevelSetImage(const unsigned int j, const InputImageType *image)
typename ListImageType::PointType ListPointType
TSingleData LevelSetDataType
typename FeatureImageType::ConstPointer FeatureImageConstPointer
A multi-dimensional iterator templated over image type that walks pixels within a region and is speci...
typename ListImageType::RegionType ListRegionType
typename InputImageType::RegionType InputRegionType
typename LevelSetDataPointerVector::iterator LevelSetDataPointerVectorIterator
typename FeatureImageType::RegionType FeatureRegionType
Helper class used to share data in the ScalarChanAndVeseLevelSetFunction.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
static constexpr unsigned int ImageDimension
TFeatureImage FeatureImageType
typename ListImageType::Pointer ListImagePointer
Templated n-dimensional image class.
LevelSetDataPointerVector m_LevelSetDataPointerVector
SmartPointer< const Self > ConstPointer
Size< VImageDimension > SizeType
typename LevelSetDataType::Pointer LevelSetDataPointer
This class generates a KdTree object without centroid information.
typename ListIndexType::IndexValueType ListIndexValueType
typename FeatureImageType::SpacingType FeatureSpacingType
void SetNumberOfNeighbors(const unsigned int n)
std::vector< LevelSetDataPointer > LevelSetDataPointerVector
unsigned long SizeValueType