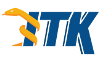 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkQuadEdgeMeshFrontIterator_h
19 #define itkQuadEdgeMeshFrontIterator_h
24 #define itkQEDefineFrontIteratorMethodsMacro(MeshTypeArg) \
28 using QEDualType = typename MeshTypeArg::QEDual; \
29 using QEPrimalType = typename MeshTypeArg::QEPrimal; \
30 using FrontDualIterator = QuadEdgeMeshFrontIterator<MeshTypeArg, QEDualType>; \
31 using ConstFrontDualIterator = QuadEdgeMeshConstFrontIterator<MeshTypeArg, QEDualType>; \
32 using FrontIterator = QuadEdgeMeshFrontIterator<MeshTypeArg, QEPrimalType>; \
33 using ConstFrontIterator = QuadEdgeMeshConstFrontIterator<MeshTypeArg, QEPrimalType>; \
35 virtual FrontIterator BeginFront(QEPrimalType * seed = (QEPrimalType *)0) \
37 return (FrontIterator(this, true, seed)); \
40 virtual ConstFrontIterator BeginFront(QEPrimalType * seed) const { return (ConstFrontIterator(this, true, seed)); } \
42 virtual FrontIterator EndFront() { return (FrontIterator(this, false)); } \
44 virtual ConstFrontIterator EndFront() const { return (ConstFrontIterator(this, false)); } \
46 virtual FrontDualIterator BeginDualFront(QEDualType * seed = (QEDualType *)0) \
48 return (FrontDualIterator(this, true, seed)); \
51 virtual ConstFrontDualIterator BeginDualFront(QEDualType * seed) const \
53 return (ConstFrontDualIterator(this, true, seed)); \
56 virtual FrontDualIterator EndDualFront() { return (FrontDualIterator(this, false)); } \
58 virtual ConstFrontDualIterator EndDualFront() const { return (ConstFrontDualIterator(this, false)); }
75 template <
typename TMesh,
typename TQE>
119 return (m_Edge == r.
m_Edge);
123 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
FrontAtom);
128 return (m_Cost < r.
m_Cost);
179 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
187 return (this->
operator++());
240 template <
typename TMesh,
typename TQE>
260 return (this->m_CurrentEdge);
271 template <
typename TMesh,
typename TQE =
typename TMesh::QEType>
308 return (this->m_CurrentEdge);
313 #include "itkQuadEdgeMeshFrontIterator.hxx"
SmartPointer< Self > Pointer
FrontType * FrontTypePointer
bool operator==(const Self &r) const
IsVisitedPointerType m_IsPointVisited
bool operator==(const FrontAtom &r) const
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
Atomic information associated to each edge of the front.
std::list< FrontAtom > FrontType
FrontAtom(QEType *e=(QEType *) 0, const CoordRepType c=0)
MeshType * GetMesh() const
QuadEdgeMeshFrontIterator(MeshType *mesh=(MeshType *) 0, bool start=true, QEType *seed=(QEType *) nullptr)
A wrapper of the STL "map" container.
typename MeshType::CoordRepType CoordRepType
typename IsVisitedContainerType::Pointer IsVisitedPointerType
virtual CoordRepType GetCost(QEType *edge)
Front iterator on Mesh class.
Non const quad edge front iterator.
FrontAtom & operator=(const FrontAtom &r)
Self & operator=(const Self &r)
const QEType * Value() const
Const quad edge mesh front iterator.
typename FrontType::iterator FrontTypeIterator
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename QEType::OriginRefType QEOriginType
static constexpr double e
QuadEdgeMeshConstFrontIterator(const MeshType *mesh=(MeshType *) 0, bool start=true, QEType *seed=(QEType *) nullptr)
Self & operator=(const NoConstType &r)