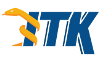 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
69 template <
typename TLabelObject>
73 ITK_DISALLOW_COPY_AND_MOVE(
LabelMap);
87 itkOverrideGetNameOfClassMacro(
LabelMap);
100 static constexpr
unsigned int ImageDimension = LabelObjectType::ImageDimension;
114 using typename Superclass::OffsetType;
128 using typename Superclass::SpacingType;
140 Initialize()
override;
144 Allocate(
bool initialize =
false)
override;
147 Graft(
const Self * imgData);
157 GetLabelObject(
const LabelType & label)
const;
235 GetLabelObject(
const IndexType & idx)
const;
275 return static_cast<SizeValueType>(m_LabelObjectContainer.size());
287 LabelObjectVectorType
288 GetLabelObjects()
const;
293 itkGetConstMacro(BackgroundValue, LabelType);
294 itkSetMacro(BackgroundValue, LabelType);
302 PrintLabelObjects(std::ostream & os)
const;
307 this->PrintLabelObjects(std::cerr);
328 m_Begin = lm->m_LabelObjectContainer.begin();
329 m_End = lm->m_LabelObjectContainer.end();
330 m_Iterator = m_Begin;
336 return m_Iterator->second;
342 return m_Iterator->first;
371 m_Iterator = m_Begin;
377 return m_Iterator == m_End;
399 m_Begin = lm->m_LabelObjectContainer.begin();
400 m_End = lm->m_LabelObjectContainer.end();
401 m_Iterator = m_Begin;
407 return m_Iterator->second;
413 return m_Iterator->first;
437 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Iterator);
442 m_Iterator = m_Begin;
448 return m_Iterator == m_End;
464 PrintSelf(std::ostream & os,
Indent indent)
const override;
467 using Superclass::Graft;
479 AddPixel(
const LabelObjectContainerIterator & it,
const IndexType & idx,
const LabelType & label);
482 RemovePixel(
const LabelObjectContainerIterator & it,
const IndexType & idx,
bool iEmitModifiedEvent);
486 #ifndef ITK_MANUAL_INSTANTIATION
487 # include "itkLabelMap.hxx"
SmartPointer< Self > Pointer
typename LabelObjectType::Pointer LabelObjectPointerType
A forward iterator over the LabelObjects of a LabelMap.
ImageBaseType::DirectionType DirectionType
bool operator==(const ConstIterator &iter) const
InternalIteratorType m_Begin
const LabelType & GetLabel() const
std::vector< LabelObjectPointerType > LabelObjectVectorType
typename LabelObjectType::LabelType LabelType
Base class for templated image classes.
A forward iterator over the LabelObjects of a LabelMap.
typename SizeType::SizeValueType SizeValueType
ImageBaseType::PointType PointType
ImageBaseType::SizeType SizeType
InternalIteratorType m_End
InternalIteratorType m_Begin
Control indentation during Print() invocation.
typename LabelObjectContainerType::const_iterator LabelObjectContainerConstIterator
ConstIterator operator++(int)
Templated n-dimensional image to store labeled objects.
typename LabelObjectContainerType::iterator LabelObjectContainerIterator
ImageBaseType::IndexType IndexType
Self::SizeValueType GetNumberOfLabelObjects() const
InternalIteratorType m_End
ConstIterator & operator++()
std::map< LabelType, LabelObjectPointerType > LabelObjectContainerType
typename std::map< LabelType, LabelObjectPointerType >::const_iterator InternalIteratorType
ImageBaseType::RegionType RegionType
void PrintLabelObjects() const
const LabelType & GetLabel() const
std::vector< LabelType > LabelVectorType
TLabelObject LabelObjectType
InternalIteratorType m_Iterator
typename std::map< LabelType, LabelObjectPointerType >::iterator InternalIteratorType
const LabelObjectType * GetLabelObject() const
Implements a weak reference to an object.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
ConstIterator(const Self *lm)
InternalIteratorType m_Iterator
Base class for most ITK classes.
bool operator==(const Iterator &iter) const
unsigned long SizeValueType
LabelObjectType * GetLabelObject()
Base class for all data objects in ITK.