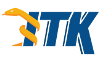 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkImageConstIteratorWithIndex_h
19 #define itkImageConstIteratorWithIndex_h
91 template <
typename TImage>
102 static constexpr
unsigned int ImageDimension = TImage::ImageDimension;
133 using AccessorFunctorType =
typename TImage::AccessorFunctorType;
157 operator=(
const Self & it);
163 return ImageDimension;
175 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
222 return m_PositionIndex;
238 m_Position = m_Image->GetBufferPointer() + m_Image->ComputeOffset(ind);
239 m_PositionIndex = ind;
247 return m_PixelAccessorFunctor.Get(*m_Position);
289 typename TImage::ConstWeakPointer m_Image{};
305 bool m_Remaining{
false };
307 AccessorType m_PixelAccessor{};
308 AccessorFunctorType m_PixelAccessorFunctor{};
312 #ifndef ITK_MANUAL_INSTANTIATION
313 # include "itkImageConstIteratorWithIndex.hxx"
bool operator>(const Self &it) const
SmartPointer< Self > Pointer
typename IndexType::IndexValueType IndexValueType
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
typename TImageType ::SizeType SizeType
bool operator<=(const Index< VDimension > &one, const Index< VDimension > &two)
const RegionType & GetRegion() const
static unsigned int GetImageDimension()
typename PixelContainer::Pointer PixelContainerPointer
typename OffsetType::OffsetValueType OffsetValueType
ImageBaseType::SizeType SizeType
const PixelType & Value() const
const IndexType & GetIndex() const
typename TImageType ::PixelType PixelType
typename TImageType ::IndexType IndexType
typename TImageType ::InternalPixelType InternalPixelType
void SetIndex(const IndexType &ind)
ImageBaseType::IndexType IndexType
bool operator>=(const Self &it) const
ImageBaseType::RegionType RegionType
const InternalPixelType * m_Position
typename TImageType ::AccessorType AccessorType
A base class for multi-dimensional iterators templated over image type that are designed to efficient...
bool IsAtReverseEnd() const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename TImageType ::RegionType RegionType
bool operator==(const Self &it) const
typename TImageType ::PixelContainer PixelContainer
typename SizeType::SizeValueType SizeValueType
unsigned long SizeValueType
typename TImageType ::OffsetType OffsetType