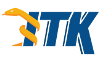 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkDeformableSimplexMesh3DGradientConstraintForceFilter_h
19 #define itkDeformableSimplexMesh3DGradientConstraintForceFilter_h
28 #include "ITKDeformableMeshExport.h"
47 ImageVoxel(
const int * pos,
const double * subpos,
double val,
double dist,
unsigned int ind)
49 this->m_Vpos[0] = pos[0];
50 this->m_Vpos[1] = pos[1];
51 this->m_Vpos[2] = pos[2];
52 this->m_Spos[0] = subpos[0];
53 this->m_Spos[1] = subpos[1];
54 this->m_Spos[2] = subpos[2];
56 this->m_Distance = dist;
99 inline friend std::ostream &
102 os <<
"Vpos: " << val.
m_Vpos << std::endl;
103 os <<
"Spos: " << val.
m_Spos << std::endl;
104 os <<
"Value: " << val.
m_Value << std::endl;
105 os <<
"Distance: " << val.
m_Distance << std::endl;
106 os <<
"Index: " << val.
m_Index << std::endl;
133 extern ITKDeformableMesh_EXPORT std::ostream &
148 template <
typename TInputMesh,
typename TOutputMesh>
174 using typename Superclass::GradientIndexType;
175 using typename Superclass::GradientIndexValueType;
176 using typename Superclass::GradientType;
177 using typename Superclass::GradientImageType;
194 itkSetMacro(Range,
int);
195 itkGetConstMacro(Range,
int);
199 #if !defined(ITK_LEGACY_REMOVE)
201 static constexpr
SIDEEnum NORMAL = SIDEEnum::NORMAL;
202 static constexpr
SIDEEnum INVERSE = SIDEEnum::INVERSE;
203 static constexpr
SIDEEnum BOTH = SIDEEnum::BOTH;
219 PrintSelf(std::ostream & os,
Indent indent)
const override;
225 ComputeExternalForce(
SimplexMeshGeometry * data,
const GradientImageType * gradientImage)
override;
234 NextVoxel(
const double *
pp,
int * ic,
double * x,
double * y,
double * z);
245 std::vector<ImageVoxel *> m_Positive{};
247 std::vector<ImageVoxel *> m_Negative{};
253 #ifndef ITK_MANUAL_INSTANTIATION
254 # include "itkDeformableSimplexMesh3DGradientConstraintForceFilter.hxx"
SmartPointer< Self > Pointer
SmartPointer< const Self > ConstPointer
typename OutputMeshType::Pointer OutputMeshPointer
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
friend std::ostream & operator<<(std::ostream &os, const ImageVoxel &val)
unsigned int GetZ() const
returns voxel Z coordinate (voxel plane)
typename InputMeshType::Pointer InputMeshPointer
ImageBaseType::PointType PointType
double GetValue() const
returns voxel value
Control indentation during Print() invocation.
handle geometric properties for vertices of a simplex mesh
ImageBaseType::IndexType IndexType
ImageVoxel(const int *pos, const double *subpos, double val, double dist, unsigned int ind)
Light weight base class for most itk classes.
double GetDistance() const
returns voxel distance to origin
unsigned int GetX() const
returns voxel X coordinate (voxel column)
void SetValue(const double val)
returns voxel position
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
unsigned int GetY() const
returns voxel Y coordinate (voxel row)
Templated n-dimensional image class.
TOutputMesh OutputMeshType
*par Constraints *The filter requires an image with at least two dimensions and a vector *length of at least The theory supports extension to scalar but *the implementation of the itk vector classes do not **The template parameter TRealType must be floating so it cannot multithread for data other than in Anisotropic Diffusion of Multivalued Images *with Application to Color IEEE Transactions on Image No pp