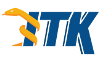 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkDefaultVectorPixelAccessor_h
19 #define itkDefaultVectorPixelAccessor_h
49 template <
typename TType>
68 InternalType * truePixel = (&output) + offset * m_OffsetMultiplier;
72 truePixel[i] = input[i];
82 return ExternalType((&input) + (offset * m_OffsetMultiplier), m_VectorLength);
90 m_OffsetMultiplier = (l - 1);
98 return m_VectorLength;
107 m_OffsetMultiplier = l - 1;
ExternalType Get(const InternalType &input, const SizeValueType offset) const
Give access to partial aspects of a type.
void Set(InternalType &output, const ExternalType &input, const unsigned long offset) const
Represents an array whose length can be defined at run-time.
void SetVectorLength(VectorLengthType l)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
unsigned int VectorLengthType
VectorLengthType GetVectorLength() const
DefaultVectorPixelAccessor(VectorLengthType l)
unsigned long SizeValueType