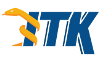 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkSymmetricSecondRankTensor_h
19 #define itkSymmetricSecondRankTensor_h
22 #ifdef SymmetricSecondRankTensor
23 # undef SymmetricSecondRankTensor
74 template <
typename TComponent,
unsigned int VDimension = 3>
83 static constexpr
unsigned int Dimension = VDimension;
84 static constexpr
unsigned int InternalDimension = VDimension * (VDimension + 1) / 2;
107 #ifdef ITK_FUTURE_LEGACY_REMOVE
117 template <
typename TCoordRepB>
130 template <
typename TCoordRepB>
134 BaseArray::operator=(pa);
141 operator=(
const ComponentType & r);
144 operator=(
const ComponentArrayType r);
155 operator+=(
const Self & r);
158 operator-=(
const Self & r);
164 operator/(
const RealValueType & r)
const;
167 operator*=(
const RealValueType & r);
170 operator/=(
const RealValueType & r);
176 return Self::InternalDimension;
183 return this->operator[](c);
190 this->operator[](c) = v;
195 operator()(
unsigned int row,
unsigned int col);
198 operator()(
unsigned int row,
unsigned int col)
const;
211 ComputeEigenValues(EigenValuesArrayType & eigenValues)
const;
216 ComputeEigenAnalysis(EigenValuesArrayType & eigenValues, EigenVectorsMatrixType & eigenVectors)
const;
221 template <
typename TMatrixValueType>
224 template <
typename TMatrixValueType>
226 Rotate(
const vnl_matrix_fixed<TMatrixValueType, VDimension, VDimension> & m)
const
230 template <
typename TMatrixValueType>
232 Rotate(
const vnl_matrix<TMatrixValueType> & m)
const
240 PreMultiply(
const MatrixType & m)
const;
244 PostMultiply(
const MatrixType & m)
const;
254 template <
typename TComponent,
unsigned int VDimension>
258 template <
typename TComponent,
unsigned int VDimension>
262 template <
typename T>
272 #ifndef ITK_MANUAL_INSTANTIATION
273 # include "itkSymmetricSecondRankTensor.hxx"
std::ostream OutputStreamType
Self Rotate(const vnl_matrix< TMatrixValueType > &m) const
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
CovariantVector< T, VVectorDimension > operator*(const T &scalar, const CovariantVector< T, VVectorDimension > &v)
ComponentType GetNthComponent(int c) const
void swap(Array< T > &a, Array< T > &b)
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
SymmetricSecondRankTensor(const SymmetricSecondRankTensor< TCoordRepB, VDimension > &pa)
Self Rotate(const vnl_matrix_fixed< TMatrixValueType, VDimension, VDimension > &m) const
Represent a symmetric tensor of second rank.
void SetNthComponent(int c, const ComponentType &v)
typename NumericTraits< ValueType >::RealType RealValueType
Self & operator=(const SymmetricSecondRankTensor< TCoordRepB, VDimension > &pa)
static unsigned int GetNumberOfComponents()
ComponentType[Self::InternalDimension] ComponentArrayType
SymmetricSecondRankTensor(const ComponentType &r)
Simulate a standard C array with copy semantics.
A templated class holding a M x N size Matrix.
typename NumericTraits< ValueType >::RealType AccumulateValueType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
std::istream InputStreamType
void swap(FixedArray &other)
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
SymmetricSecondRankTensor(const ComponentArrayType r)
constexpr unsigned int Dimension
std::istream & operator>>(std::istream &is, Point< T, VPointDimension > &vct)