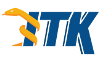 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkShapeLabelObjectAccessors_h
19 #define itkShapeLabelObjectAccessors_h
37 template <
typename TLabelObject>
47 return labelObject->GetNumberOfPixels();
51 template <
typename TLabelObject>
61 return labelObject->GetBoundingBox();
65 template <
typename TLabelObject>
75 return labelObject->GetPhysicalSize();
79 template <
typename TLabelObject>
89 return labelObject->GetNumberOfPixelsOnBorder();
93 template <
typename TLabelObject>
103 return labelObject->GetPerimeterOnBorder();
107 template <
typename TLabelObject>
117 return labelObject->GetCentroid();
121 template <
typename TLabelObject>
131 return labelObject->GetFeretDiameter();
135 template <
typename TLabelObject>
145 return labelObject->GetPrincipalMoments();
149 template <
typename TLabelObject>
159 return labelObject->GetPrincipalAxes();
163 template <
typename TLabelObject>
173 return labelObject->GetElongation();
177 template <
typename TLabelObject>
187 return labelObject->GetPerimeter();
191 template <
typename TLabelObject>
201 return labelObject->GetRoundness();
205 template <
typename TLabelObject>
215 return labelObject->GetEquivalentSphericalRadius();
219 template <
typename TLabelObject>
229 return labelObject->GetEquivalentSphericalPerimeter();
233 template <
typename TLabelObject>
243 return labelObject->GetEquivalentEllipsoidDiameter();
247 template <
typename TLabelObject>
257 return labelObject->GetFlatness();
261 template <
typename TLabelObject>
271 return labelObject->GetPerimeterOnBorderRatio();
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
typename LabelObjectType::MatrixType AttributeValueType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
double AttributeValueType
ImageBaseType::SpacingType VectorType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
typename LabelObjectType::VectorType AttributeValueType
SizeValueType AttributeValueType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
double AttributeValueType
SizeValueType AttributeValueType
double AttributeValueType
typename LabelObjectType::CentroidType AttributeValueType
double AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
ImageBaseType::RegionType RegionType
TLabelObject LabelObjectType
double AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
TLabelObject LabelObjectType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
TLabelObject LabelObjectType
double AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
double AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
double AttributeValueType
TLabelObject LabelObjectType
double AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
TLabelObject LabelObjectType
typename LabelObjectType::RegionType AttributeValueType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
typename LabelObjectType::VectorType AttributeValueType
TLabelObject LabelObjectType
unsigned long SizeValueType
double AttributeValueType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const