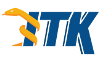 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
57 template <
typename TComponent =
unsigned short>
72 static constexpr
unsigned int Length = 3;
80 #ifdef ITK_FUTURE_LEGACY_REMOVE
87 #if defined(ITK_LEGACY_REMOVE)
97 RGBPixel(
const ComponentType & r) { this->Fill(r); }
101 template <
typename TRGBPixelValueType>
111 template <
typename TRGBPixelValueType>
115 BaseArray::operator=(r);
121 operator=(
const ComponentType r[3]);
130 operator/(
const ComponentType & r)
const;
135 operator+=(
const Self & r);
137 operator-=(
const Self & r);
139 operator*=(
const ComponentType & r);
141 operator/=(
const ComponentType & r);
162 return this->operator[](c);
169 return static_cast<ComponentType>(
170 std::sqrt(static_cast<double>(this->
operator[](0)) * static_cast<double>(this->
operator[](0)) +
171 static_cast<double>(this->
operator[](1)) * static_cast<double>(this->
operator[](1)) +
172 static_cast<double>(this->
operator[](2)) * static_cast<double>(this->
operator[](2))));
179 this->operator[](c) = v;
186 this->operator[](0) = red;
193 this->operator[](1) = green;
200 this->operator[](2) = blue;
207 this->operator[](0) = red;
208 this->operator[](1) = green;
209 this->operator[](2) = blue;
214 const ComponentType &
217 return this->operator[](0);
221 const ComponentType &
224 return this->operator[](1);
228 const ComponentType &
231 return this->operator[](2);
236 GetLuminance()
const;
239 template <
typename TComponent>
241 operator<<(std::ostream & os,
const RGBPixel<TComponent> & c);
243 template <
typename TComponent>
245 operator>>(std::istream & is, RGBPixel<TComponent> & c);
247 template <
typename T>
267 #ifndef ITK_MANUAL_INSTANTIATION
268 # include "itkRGBPixel.hxx"
static unsigned int GetNumberOfComponents()
Represent Red, Green and Blue components for color images.
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
const ComponentType & GetRed() const
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
CovariantVector< T, VVectorDimension > operator*(const T &scalar, const CovariantVector< T, VVectorDimension > &v)
void Set(ComponentType red, ComponentType green, ComponentType blue)
typename NumericTraits< ComponentType >::RealType LuminanceType
Self & operator=(const RGBPixel< TRGBPixelValueType > &r)
void swap(Array< T > &a, Array< T > &b)
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
RGBPixel(const ComponentType &r)
const ComponentType & GetGreen() const
ComponentType GetScalarValue() const
const ComponentType & GetBlue() const
RGBPixel(const ComponentType r[3])
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
Simulate a standard C array with copy semantics.
void SetGreen(ComponentType green)
RGBPixel(const RGBPixel< TRGBPixelValueType > &r)
void SetNthComponent(int c, const ComponentType &v)
ComponentType GetNthComponent(int c) const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void swap(FixedArray &other)
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
void SetRed(ComponentType red)
constexpr unsigned int Dimension
void SetBlue(ComponentType blue)
std::istream & operator>>(std::istream &is, Point< T, VPointDimension > &vct)