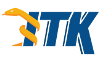 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
25 #include "vnl/vnl_vector_ref.h"
52 template <
typename TCoordRep,
unsigned int VPo
intDimension = 3>
69 static constexpr
unsigned int PointDimension = VPointDimension;
80 return VPointDimension;
91 template <
typename TPo
intValueType>
97 template <
typename TPo
intValueType>
98 Point(
const TPointValueType r[VPointDimension])
106 #if defined(ITK_LEGACY_REMOVE)
108 Point(std::nullptr_t) =
delete;
111 template <
typename TPo
intValueType>
112 explicit Point(
const TPointValueType & v)
122 template <
typename TPo
intValueType>
123 Point(
const TPointValueType & v)
126 Point(
const ValueType & v)
133 explicit Point(
const std::array<ValueType, VPointDimension> & stdArray)
139 operator=(
const ValueType r[VPointDimension]);
148 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Self);
172 GetVectorFromOrigin()
const;
175 vnl_vector_ref<TCoordRep>
179 vnl_vector<TCoordRep>
180 GetVnlVector()
const;
194 SetToMidPoint(
const Self &,
const Self &);
223 SetToBarycentricCombination(
const Self & A,
const Self & B,
double alpha);
242 SetToBarycentricCombination(
const Self & A,
const Self & B,
const Self & C,
double weightForA,
double weightForB);
258 SetToBarycentricCombination(
const Self * P,
const double * weights,
unsigned int N);
263 template <
typename TCoordRepB>
267 for (
unsigned int i = 0; i < VPointDimension; ++i)
269 (*this)[i] = static_cast<TCoordRep>(pa[i]);
278 template <
typename TCoordRepB>
284 for (
unsigned int i = 0; i < VPointDimension; ++i)
286 const auto component = static_cast<RealType>(pa[i]);
287 const RealType difference = static_cast<RealType>((*
this)[i]) - component;
288 sum += difference * difference;
296 template <
typename TCoordRepB>
300 const double distance = std::sqrt(static_cast<double>(this->SquaredEuclideanDistanceTo(pa)));
302 return static_cast<RealType>(distance);
306 template <
typename T,
unsigned int VPo
intDimension>
308 operator<<(std::ostream & os,
const Point<T, VPointDimension> & vct);
310 template <
typename T,
unsigned int VPo
intDimension>
312 operator>>(std::istream & is, Point<T, VPointDimension> & vct);
340 template <
typename TPo
intContainer,
typename TWeightContainer>
356 template <
typename TCoordRep,
unsigned int VPo
intDimension>
365 template <
typename TValue,
typename... TVariadic>
367 MakePoint(
const TValue firstValue,
const TVariadic... otherValues)
369 static_assert(std::conjunction_v<std::is_same<TVariadic, TValue>...>,
370 "The other values should have the same type as the first value.");
372 constexpr
unsigned int dimension{ 1 +
sizeof...(TVariadic) };
373 const std::array<TValue, dimension> stdArray{ { firstValue, otherValues... } };
379 #ifndef ITK_MANUAL_INSTANTIATION
380 # include "itkPoint.hxx"
SmartPointer< Self > Pointer
auto MakePoint(const TValue firstValue, const TVariadic... otherValues)
typename NumericTraits< ValueType >::RealType RealType
Point(const TPointValueType r[VPointDimension])
Computes the barycentric combination of an array of N points.
Point(const TPointValueType &v)
TWeightContainer WeightContainerType
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
ImageBaseType::SpacingType VectorType
bool operator==(const Self &pt) const
void swap(Array< T > &a, Array< T > &b)
A templated class holding a n-Dimensional vector.
ConstNeighborhoodIterator< TImage > operator-(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
static unsigned int GetPointDimension()
const ValueType * ConstIterator
void CastFrom(const Point< TCoordRepB, VPointDimension > &pa)
Point(const ValueType r[VPointDimension])
RealType EuclideanDistanceTo(const Point< TCoordRepB, VPointDimension > &pa) const
typename PointContainerType::Element PointType
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
RealType SquaredEuclideanDistanceTo(const Point< TCoordRepB, VPointDimension > &pa) const
Simulate a standard C array with copy semantics.
Point(const ValueType &v)
Point(const std::array< ValueType, VPointDimension > &stdArray)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename PointContainerType::Pointer PointContainerPointer
void swap(FixedArray &other)
ConstNeighborhoodIterator< TImage > operator+(const ConstNeighborhoodIterator< TImage > &it, const typename ConstNeighborhoodIterator< TImage >::OffsetType &ind)
A templated class holding a geometric point in n-Dimensional space.
TPointContainer PointContainerType
std::istream & operator>>(std::istream &is, Point< T, VPointDimension > &vct)
Point(const Point< TPointValueType, VPointDimension > &r)