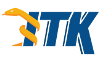 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkNotImageFilter_h
19 #define itkNotImageFilter_h
55 template <
typename TInputImage,
typename TOutputImage>
59 Functor::NOT<typename TInputImage::PixelType, typename TOutputImage::PixelType>>
92 typename TOutputImage::PixelType
106 <<
" foregroundValue: " << foregroundValue << std::endl;
113 typename TOutputImage::PixelType
121 #ifdef ITK_USE_CONCEPT_CHECKING
Implements pixel-wise generic operation on one image.
TOutput GetForegroundValue() const
void SetBackgroundValue(const typename TOutputImage::PixelType &backgroundValue)
Unary logical NOT functor.
bool NotExactlyEquals(const TInput1 &x1, const TInput2 &x2)
TOutputImage::PixelType GetForegroundValue() const
void SetForegroundValue(const TOutput &FG)
Base class for all process objects that output image data.
~NotImageFilter() override=default
TOutputImage::PixelType GetBackgroundValue() const
#define itkConceptMacro(name, concept)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
FunctorType & GetFunctor()
TOutput GetBackgroundValue() const
void SetForegroundValue(const typename TOutputImage::PixelType &foregroundValue)
virtual void Modified() const
Implements the NOT logical operator pixel-wise on an image.
void SetBackgroundValue(const TOutput &BG)