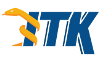 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkLevelSetEquationTermContainer_h
20 #define itkLevelSetEquationTermContainer_h
28 #include <unordered_map>
41 template <
typename TInputImage,
typename TLevelSetContainer>
105 GetTerm(
const std::string & iName);
119 InitializeParameters();
134 ComputeCFLContribution()
const;
157 : m_Iterator(it.m_Iterator)
193 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Iterator);
206 return m_Iterator->first;
212 return m_Iterator->second;
228 : m_Iterator(it.m_Iterator)
268 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Iterator);
281 return m_Iterator->first;
287 return m_Iterator->second;
332 #ifndef ITK_MANUAL_INSTANTIATION
333 # include "itkLevelSetEquationTermContainer.hxx"
336 #endif // itkLevelSetEquationTermContainer_h
SmartPointer< Self > Pointer
std::map< TermIdType, std::atomic< LevelSetOutputRealType > > MapCFLContainerType
std::unordered_set< std::string > RequiredDataType
typename InputImageType::Pointer InputImagePointer
MapTermContainerConstIteratorType m_Iterator
bool operator==(const Iterator &it) const
typename LevelSetContainerType::LevelSetPointer LevelSetPointer
bool operator==(const ConstIterator &it) const
ConstIterator(const MapTermContainerConstIteratorType &it)
ConstIterator operator++(int)
typename LevelSetContainerType::LevelSetDataType LevelSetDataType
Iterator(const ConstIterator &it)
ConstIterator & operator*()
typename LevelSetContainerType::InputIndexType LevelSetInputIndexType
typename LevelSetContainerType::Pointer LevelSetContainerPointer
ConstIterator operator--(int)
ConstIterator & operator--()
ConstIterator(const Iterator &it)
typename LevelSetContainerType::HessianType LevelSetHessianType
Light weight base class for most itk classes.
typename LevelSetContainerType::OutputRealType LevelSetOutputRealType
ConstIterator * operator->()
TermIdType GetIdentifier() const
typename MapCFLContainerType::iterator MapCFLContainerIterator
std::unordered_map< std::string, TermPointer > HashMapStringTermContainerType
TermType * GetTerm() const
TermIdType GetIdentifier() const
TInputImage InputImageType
typename LevelSetContainerType::GradientType LevelSetGradientType
TLevelSetContainer LevelSetContainerType
Iterator(const MapTermContainerIteratorType &it)
typename MapCFLContainerType::const_iterator MapCFLContainerConstIterator
typename MapTermContainerType::const_iterator MapTermContainerConstIteratorType
typename LevelSetContainerType::OutputType LevelSetOutputPixelType
bool operator==(const ConstIterator &it) const
Abstract class to represents a term in the level-set evolution PDE.
typename LevelSetContainerType::LevelSetIdentifierType LevelSetIdentifierType
TermType * GetTerm() const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Base class for most ITK classes.
typename TermType::Pointer TermPointer
MapTermContainerIteratorType m_Iterator
typename TermType::RequiredDataType RequiredDataType
typename MapTermContainerType::iterator MapTermContainerIteratorType
std::map< TermIdType, TermPointer > MapTermContainerType
bool operator==(const Iterator &it) const
Class for container holding the terms of a given level set update equation.
typename LevelSetContainerType::LevelSetType LevelSetType
ConstIterator & operator++()