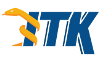 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
28 #ifndef itkImageToImageFilterDetail_h
29 #define itkImageToImageFilterDetail_h
44 namespace ImageToImageFilterDetail
95 template <
unsigned int>
107 template <
bool B1,
bool B2>
123 template <
int D1,
int D2>
144 template <
unsigned int D1,
unsigned int D2>
190 template <
unsigned int D1,
unsigned int D2>
196 destRegion = srcRegion;
215 template <
unsigned int D1,
unsigned int D2>
231 for (dim = 0; dim < D1; ++dim)
233 destIndex[dim] = srcIndex[dim];
234 destSize[dim] = srcSize[dim];
257 template <
unsigned int D1,
unsigned int D2>
273 for (dim = 0; dim < D2; ++dim)
275 destIndex[dim] = srcIndex[dim];
276 destSize[dim] = srcSize[dim];
279 for (; dim < D1; ++dim)
327 template <
unsigned int D1,
unsigned int D2>
335 ImageToImageFilterDefaultCopyRegion<D1, D2>(ComparisonType(), destRegion, srcRegion);
343 template <
unsigned int D1,
unsigned int D2>
353 template <
unsigned int D1,
unsigned int D2>
361 template <
unsigned int D1,
unsigned int D2>
371 template <
unsigned int D1,
unsigned int D2>
383 const typename SourceImageType::SpacingType & inputSpacing = srcImage->
GetSpacing();
387 typename DestinationImageType::SpacingType destSpacing;
394 for (; i < SourceImageType::ImageDimension; ++i)
396 destSpacing[i] = inputSpacing[i];
397 destOrigin[i] = inputOrigin[i];
398 for (
unsigned int j = 0; j < DestinationImageType::ImageDimension; ++j)
400 if (j < SourceImageType::ImageDimension)
402 destDirection[j][i] = inputDirection[j][i];
406 destDirection[j][i] = 0.0;
410 for (; i < DestinationImageType::ImageDimension; ++i)
412 destSpacing[i] = 1.0;
414 for (
unsigned int j = 0; j < DestinationImageType::ImageDimension; ++j)
418 destDirection[j][i] = 1.0;
422 destDirection[j][i] = 0.0;
447 template <
unsigned int D1,
unsigned int D2>
455 ImageToImageFilterDefaultCopyInformation<D1, D2>(ComparisonType(), destImage, srcImage);
virtual unsigned int GetNumberOfComponentsPerPixel() const
Represent a n-dimensional index in a n-dimensional image.
ImageBaseType::DirectionType DirectionType
Represent a n-dimensional size (bounds) of a n-dimensional image.
Templated class to produce a unique type for each unsigned integer (usually a dimension).
bool operator!=(const ImageRegionCopier< D1, D2 > &c1, const ImageRegionCopier< D1, D2 > &c2)
Base class for templated image classes.
const IndexType & GetIndex() const
An image region represents a structured region of data.
ImageBaseType::PointType PointType
void CopyInformation(const DataObject *data) override
void ImageToImageFilterDefaultCopyInformation(const typename BinaryUnsignedIntDispatch< D1, D2 >::FirstEqualsSecondType &, ImageBase< D1 > *destImage, const ImageBase< D2 > *srcImage)
Templated class to produce a unique type "true" and "false".
virtual const PointType & GetOrigin() const
const SizeType & GetSize() const
Templated class to produce a unique type for a pairing of unsigned integers (usually two dimensions).
void ImageToImageFilterDefaultCopyRegion(const typename BinaryUnsignedIntDispatch< D1, D2 >::FirstEqualsSecondType &, ImageRegion< D1 > &destRegion, const ImageRegion< D2 > &srcRegion)
Base class for a class used to dispatch to dimension specific implementations.
virtual void SetDirection(const DirectionType &direction)
virtual void operator()(ImageRegion< D1 > &destRegion, const ImageRegion< D2 > &srcRegion) const
Templated class to produce a unique type for a pairing of integers.
Templated class to produce a unique type for each integer.
Templated class to produce a unique type for a pairing of booleans.
virtual void SetOrigin(PointType _arg)
virtual const SpacingType & GetSpacing() const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
virtual const DirectionType & GetDirection() const
virtual void SetSpacing(const SpacingType &spacing)
void SetIndex(const IndexType &index)
std::ostream & operator<<(std::ostream &os, const ImageRegionCopier< D1, D2 > &)
virtual void SetNumberOfComponentsPerPixel(unsigned int)
A Function object used to dispatching to a routine to copy a region (start index and size).
void SetSize(const SizeType &size)
virtual ~ImageRegionCopier()=default