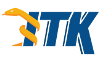 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkDefaultConvertPixelTraits_h
19 #define itkDefaultConvertPixelTraits_h
40 template <
typename PixelType>
51 return PixelType::GetNumberOfComponents();
57 return PixelType::GetNumberOfComponents();
64 return pixel.GetNthComponent(c);
71 pixel.SetNthComponent(c, v);
78 return pixel.GetScalarValue();
83 #define ITK_DEFAULTCONVERTTRAITS_NATIVE_SPECIAL(type) \
85 class ITK_TEMPLATE_EXPORT DefaultConvertPixelTraits<type> \
88 using ComponentType = type; \
90 GetNumberOfComponents() \
95 GetNumberOfComponents(const type) \
100 SetNthComponent(int, type & pixel, const ComponentType & v) \
105 GetNthComponent(int, const type pixel) \
110 GetScalarValue(const type & pixel) \
132 #undef ITK_DEFAULTCONVERTTRAITS_NATIVE_SPECIAL
138 template <
unsigned int VDimension>
165 #define ITK_DEFAULTCONVERTTRAITS_FIXEDARRAY_TYPE(type) \
166 template <typename TComponentType, unsigned int VDimension> \
167 class ITK_TEMPLATE_EXPORT DefaultConvertPixelTraits<type<TComponentType, VDimension>> \
170 using TargetType = type<TComponentType, VDimension>; \
171 using ComponentType = TComponentType; \
172 static unsigned int \
173 GetNumberOfComponents() \
177 static unsigned int \
178 GetNumberOfComponents(const TargetType) \
183 SetNthComponent(int i, TargetType & pixel, const ComponentType & v) \
187 static ComponentType \
188 GetNthComponent(int i, const TargetType pixel) \
192 static ComponentType \
193 GetScalarValue(const TargetType & pixel) \
212 template <
typename VComponent>
249 template <
typename VComponent>
268 const unsigned int row = i / pixel.
Cols();
269 const unsigned int col = i % pixel.
Cols();
275 const unsigned int row = i / pixel.
Cols();
276 const unsigned int col = i % pixel.
Cols();
277 return pixel(row, col);
296 template <
typename VComponent,
unsigned int VRows,
unsigned int VCols>
305 return VRows * VCols;
310 const unsigned int row = i / VCols;
311 const unsigned int col = i % VCols;
317 const unsigned int row = i / VCols;
318 const unsigned int col = i % VCols;
319 return pixel[row][col];
332 template <
typename TComponent>
358 return std::norm(pixel);
static void SetNthComponent(int i, TargetType &pixel, const ComponentType &v)
static unsigned int GetNumberOfComponents()
typename TargetType::OffsetValueType ComponentType
static void SetNthComponent(int i, TargetType &pixel, const ComponentType &v)
static ComponentType GetScalarValue(const TargetType &pixel)
static void SetNthComponent(int i, TargetType &pixel, const ComponentType &v)
static void SetNthComponent(int i, TargetType &pixel, const ComponentType &v)
static unsigned int GetNumberOfComponents()
static unsigned int GetNumberOfComponents(const PixelType)
static ComponentType GetNthComponent(int i, const TargetType &pixel)
static ComponentType GetScalarValue(const TargetType &pixel)
static unsigned int GetNumberOfComponents()
RealValueType GetNorm() const
static unsigned int GetNumberOfComponents()
static ComponentType GetScalarValue(const TargetType &)
std::complex< TComponent > TargetType
A templated class holding a M x N size Matrix.
static ComponentType GetScalarValue(const TargetType &pixel)
static unsigned int GetNumberOfComponents(const TargetType pixel)
static unsigned int GetNumberOfComponents()
static unsigned int GetNumberOfComponents(const TargetType pixel)
static ComponentType GetScalarValue(const PixelType &pixel)
Traits class used to by ConvertPixels to convert blocks of pixels.
static void SetNthComponent(int c, PixelType &pixel, const ComponentType &v)
unsigned int Cols() const
static ComponentType GetNthComponent(int i, const TargetType &pixel)
unsigned int Size() const
ITK_DEFAULTCONVERTTRAITS_FIXEDARRAY_TYPE(Vector)
Represents an array whose length can be defined at run-time.
A templated class holding a M x N size Matrix.
Represent a n-dimensional offset between two n-dimensional indexes of n-dimensional image.
static void SetNthComponent(int i, TargetType &pixel, const ComponentType &v)
ITK_DEFAULTCONVERTTRAITS_NATIVE_SPECIAL(char)
static unsigned int GetNumberOfComponents()
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
static ComponentType GetNthComponent(int c, const PixelType &pixel)
unsigned int Rows() const
static ComponentType GetNthComponent(int i, const TargetType &pixel)
static ComponentType GetScalarValue(const TargetType &pixel)
typename PixelType::ComponentType ComponentType